● location 객체
- 현재 브라우저에 표시된 HTML문서의 주소를 얻거나, 브라우저에 새 문서를 불러올 때 사용할 수 있다.
- location 객체의 프로퍼티와 메소드를 이용하면, 현재 문서의 URL 주소를 다양하게 해석하여 처리할 수 있게 된다.
1. href
- 페이지의 URL 전체 정보 반환. URL을 지정하여 페이지 이동 가능하다.
2. pathname
- URL 경로부분의 정보를 반환한다.
3. port
- 포트번호를 반환한다.
4. reload()
- 새로 고침
5. assign()
- 현재 URL을 지정한 URL로 바꿔서 페이지 이동
6. replace()
- 현재 URL을 지정한 URL로 바꾸고 이전 페이지로 돌아갈 수 없게 한다.
● history 객체
- 브라우저의 히스토리 정보를 문서와 문서 상태 목록으로 저장하는 객체이다.
1. go(n) : n번째로 이동(n은 0부터 시작)
- n이 양수일 때 : 앞으로 가기
- n이 음수일 때 : 뒤로가기
2. back() : 뒤 페이지로 이동
3. forward() : 앞 페이지로 이동
실습(window)
1. checkbox 클릭 시 팝업창 닫힘
- window1.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>메인 화면</title>
</head>
<body>
</body>
<script>
window.open("window2.html", "_blank", "width=490, height=730");
// with(console){
// log("현재 문서의 호스트 이름은 " + location.hostname + "입니다.");
// log("현재 문서의 URL 주소는 " + location.href + "입니다.");
// log("현재 문서의 경로는 " + location.pathname + "입니다.");
// }
</script>
</html>
- window2.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>팝업창</title>
<style>
* {
margin: 0;
}
html {
overflow: hidden; /* 스크롤을 생성하지 않는다. */
}
div {
text-align: right;
padding-right: 15px;
}
div span {
cursor: pointer;
}
</style>
</head>
<body>
<div>
<label>
<input type="checkbox" value="ok">
<span>오늘 하루동안 이 창을 열지 않기</span>
</label>
</div>
</body>
<script>
let checkbox = document.querySelector("input[type='checkbox']");
checkbox.addEventListener('click', function(){
let check = checkbox.checked;
if(check){
window.close();
}
})
</script>
</html>
2. 링크 클릭 시 상세 페이지 출력
- window1.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>메인 화면</title>
</head>
<body>
</body>
<script>
window.open("window2.html", "_blank", "width=490, height=730");
</script>
</html>
- window2.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>팝업창</title>
</head>
<body>
<a href="javascript:opener.parent.location='window3.html';window.close();">
<img src="img/팝업.png">
</a>
</html>
-window3.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>상세 페이지</title>
<style>
div#img {
background-image: url("img/이벤트.png");
background-size: 100% 100%;
background-position: center;
width: 891px;
height: 2520px;
}
</style>
</head>
<body>
<div id="img"></div>
</body>
</html>
실습(textarea)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>textarea</title>
</head>
<body>
<textarea name="" id="" cols="30" rows="10">안녕하세요</textarea>
</body>
</html>
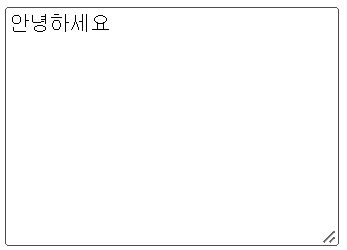
실습(location)
- 문제
<!--
네이버, 다음, 구글, 3가지 사이트를 사용자가 골라서 이동할 수 있는 페이지를 제작한다.
선택한 사이트를 location객체를 사용하여, 이동시킨다.
radio버튼 3개를 구현해야하며, 모두 동일한 name을 작성한다.
JS에서 name으로 해당 radio객체를 가져오면 선택한 value를 가져올 수 있다.
form태그 안에 radio버튼을 제작하고, form객체를 통해 input태그를 가져온다.
value 프로퍼티를 사용하여 사용자가 선택한 사이트를 구분한다.
이동 버튼을 클릭하면 선택한 사이트로 이동할 수 있게 구현한다.
location.href = "이동할 URL";
-->
- 코드
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>사이트 이동</title>
</head>
<body>
<form action="" name="myForm">
<label>
네이버
<input type="radio" name="site" value="naver">
</label>
<label>
다음
<input type="radio" name="site" value="daum">
</label>
<label>
구글
<input type="radio" name="site" value="google">
</label>
<div>
<input type="button" value="이동" onclick="send()">
</div>
</form>
</body>
<script>
function send(){
let url = "";
if(!myForm.site.value){
alert("사이트를 선택해주세요.");
}
switch(myForm.site.value){
case 'naver':
url = "https://www.naver.com";
break;
case 'daum':
url = "https://www.daum.net";
break;
case 'google':
url = "https://www.google.com";
break;
}
location.href = url;
}
</script>
</html>
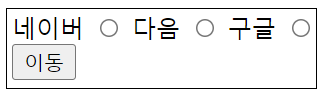
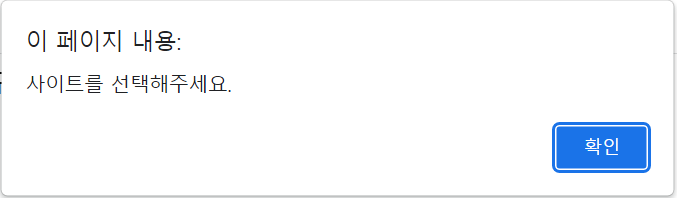
실습(history)
- locationTest2.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>히스토리 목록의 개수</title>
</head>
<body>
<h1>히스토리 목록의 개수</h1>
<p>아래 버튼을 눌러 새로운 문서를 연 후, 브라우저의 뒤로가기 버튼을 눌러보세요!</p>
<button onclick="move()">새로운 문서 열기</button>
<button onclick="history.forward()">앞으로</button>
<p id="text"></p>
</body>
<script>
function move(){
location.assign("historyTest.html");
// location.replace("historyTest.html");
}
document.getElementById("text").innerHTML =
"현재 브라우저의 히스토리 목록의 개수는 " + history.length + "개 입니다.";
</script>
</html>
- historyTest.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>history 객체 예제</title>
</head>
<body>
<h1>히스토리 목록의 접근</h1>
<p>아래 버튼을 눌러보세요.</p>
<button onclick="history.back()">뒤로</button>
<button onclick="history.go(-1)">원하는 곳으로 이동</button>
</body>
</html>
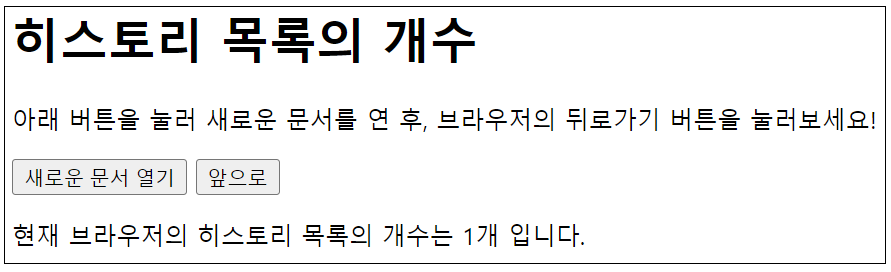

'웹 개발 > JavaScript' 카테고리의 다른 글
[Web_JavaScript] 18 (0) | 2022.05.14 |
---|---|
[Web_JavaScript] 17 (0) | 2022.05.13 |
[Web_JavaScript] 15 (0) | 2022.05.11 |
[Web_JavaScript] 14 (0) | 2022.05.10 |
[Web_JavaScript] 13 (0) | 2022.05.09 |