실습(event)
- 첨부파일 썸네일
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>첨부파일 썸네일</title>
<style>
label[for='attach'] div{
width: 150px;
height: 150px;
background-image: url('img/attach.png');
background-size: 100% 100%;
cursor: pointer;
}
</style>
</head>
<body>
<label for="attach">
<div></div>
</label>
<input type="file" id="attach" style="display:none">
</body>
<script>
const file = document.querySelector("input[type='file']");
const thumbnail = document.querySelector("label[for='attach'] div");
// file 타입의 input 태그에 파일을 업로드할 때 마다, change 이벤트가 발생한다.
// change 이벤트가 발생한 객체에 대한 정보는 event 매개변수로 전달받는다.
file.addEventListener("change", function(event){
let reader = new FileReader();
reader.readAsDataURL(event.target.files[0]);
reader.onload = function(event){
let url = event.target.result;
if(url.includes("image")){
thumbnail.style.backgroundImage = "url('" + url +"')";
}else{
thumbnail.style.backgroundImage = "url('img/attach.png')";
}
}
});
</script>
</html>

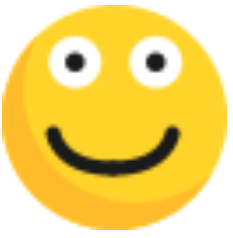
실습(DOM 정리)
- 아래 이미지처럼 출력되는 프로그램
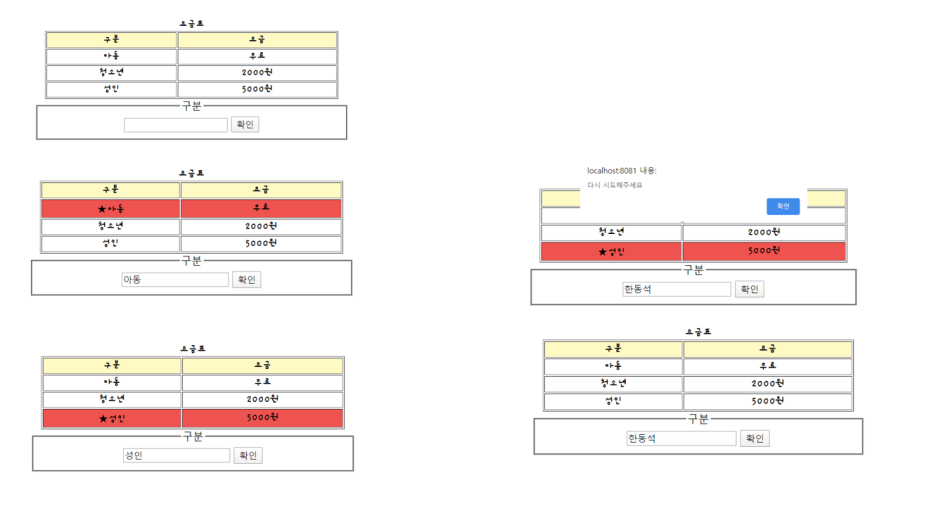
1. css
body {
font-family: 'Jua', sans-serif;
font-size: 1.5rem;
}
table{
width: 30%;
text-align: center;
margin: 30px auto;
}
fieldset{
text-align: center;
width: 25.5%;
margin: 0 auto;
}
thead{
background-color: #fff9c4;
}
2. html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Jua&display=swap" rel="stylesheet">
<link rel="stylesheet" href="task01.css">
<title>요금표</title>
</head>
<body>
<table border="1">
<caption>요금표</caption>
<thead>
<tr>
<th>구분</th>
<th>요금</th>
</tr>
</thead>
<tbody>
<tr>
<td>아동</td>
<td>무료</td>
</tr>
<tr>
<td>청소년</td>
<td>2000원</td>
</tr>
<tr>
<td>성인</td>
<td>5000원</td>
</tr>
</tbody>
</table>
<fieldset>
<legend>구분</legend>
<input type="text" id="input">
<button onclick="confirm()">확인</button>
</fieldset>
</body>
<script src="task01.js"></script>
</html>
3. js
let tempTr;
let tempText;
function confirm() {
const input = document.getElementById("input").value; // 사용자가 입력한 값
const trs = document.querySelectorAll("tbody tr"); // tr 3개
let check = false;
if(tempTr) {
tempTr.style.background = "#fff";
tempTr.firstElementChild.innerHTML = tempText;
}
trs.forEach(tr => {
let td = tr.firstElementChild;
if(td.innerHTML == input) {
tempTr = tr;
tempText = td.innerHTML;
tr.style.background = "#ef5350";
td.innerHTML = "★" + input;
check = true;
}
});
if(!check) {
alert("다시 시도해주세요.");
}
}

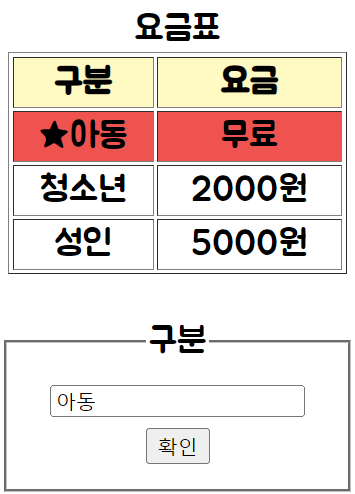
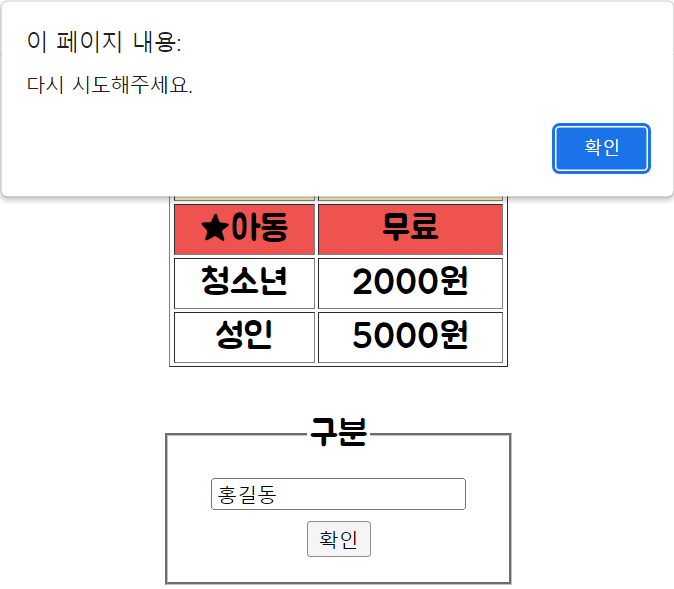
'웹 개발 > JavaScript' 카테고리의 다른 글
[Web_JavaScript] 15 (0) | 2022.05.11 |
---|---|
[Web_JavaScript] 14 (0) | 2022.05.10 |
[Web_JavaScript] 12 (0) | 2022.05.08 |
[Web_JavaScript] 11 (0) | 2022.05.07 |
[Web_JavaScript] 10 (0) | 2022.05.06 |