● GET 방식과 POST 방식
1. GET
- 주소에 데이터를 추기하여 전달하는 방식
- 보통 쿼리 문자열(query string)에 포함되어 전송되므로 길이에 제한이 있으며
주소에 데이터가 보이므로 보안상 취약점이 존재한다.
- 하지만 GET 방식이 POST 방식보다 상대적으로 빠른 전송방식이다.
2. POST
- 데이터를 별도로 첨부(Header)하여 전달하는 방식
- 브라우저 히스토리에 남지 않고 데이터는 쿼리 문자열과는 별도로 전송된다.
- 따라서 데이터의 길이에 제한도 없으며, GET 방식보다는 보안성이 높다.
- 하지만 GET 방식보다 상대적으로 느리다.
※ 결론
- GET 방식: 전송할 데이터의 양이 적고 노출되어도 무방하다면 사용한다.
- POST 방식: 전송할 데이터의 양이 많거나 노출이 되면 안될 데이터라면 사용한다.
실습(input)
- 문제
<!--
inputAge.jsp에서 나이를 입력받고 다시 inputName.jsp로 온다.
이 때 inputName.jsp에서 age파라미터의 값이 null이 아니라면 나이를 입력받고 돌아온 것이다.
만약 age까지 모두 입력받았다면 inputName.jsp페이지에서 이름과 나이를 출력한다.
-->
1. inputAge.jsp
<%@page import="java.net.URLEncoder"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>데이터 전송 실습</title>
</head>
<body>
<%-- <form action="inputName.jsp">
<input type="hidden" name="name" value="<%=request.getParameter("name")%>">
<input type="text" name="age" placeholder="나이">
<button>전송</button>
</form> --%>
<%
response.sendRedirect("inputName.jsp?name=" + URLEncoder.encode(request.getParameter("name"), "UTF-8") + "&age=20");
%>
</body>
</html>
2. inputName.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>데이터 전송 실습</title>
</head>
<body>
<%
//선언문에서는 내장객체를 사용할 수 없다.
String name = request.getParameter("name");
String age = request.getParameter("age");
%>
<%if(age == null){%>
<form action="inputAge.jsp">
<input type="text" name="name" placeholder="이름">
<input type="submit" value="전송">
</form>
<%}else{ %>
<h1>이름 : <%=name %></h1>
<h1>나이 : <%=age %>살</h1>
<%} %>
</body>
</html>
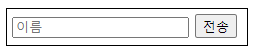

실습(GET방식과 POST 방식)
1. postTest.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>POST 방식의 요청</title>
</head>
<body>
<h1>POST 방식의 요청</h1>
<form action="getTest.jsp" method="post">
<input type="text" value="Seoul" name="city" readonly>
<input type="text" value="15155" name="zipcode" readonly>
<button>POST 방식으로 요청 보내기!</button>
</form>
</body>
</html>
2. getTest.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>GET 방식의 요청</title>
</head>
<body>
<%
String city = request.getParameter("city");
String zipcode = request.getParameter("zipcode");
if(city == null || zipcode == null){
response.sendRedirect("postTest.jsp");
}
%>
<h1>GET 방식의 요청</h1>
<form>
<input type="text" value="Seoul" name="city" readonly>
<input type="text" value="15155" name="zipcode" readonly>
<button>GET 방식으로 요청 보내기!</button>
</form>
<p>
<%=city %>
<%=zipcode %>
</p>
</body>
</html>

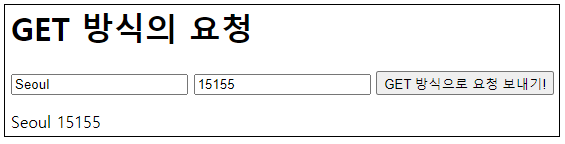
'웹 개발 > JSP' 카테고리의 다른 글
[Web_JSP] 08 (0) | 2022.05.27 |
---|---|
[Web_JSP] 07 (0) | 2022.05.26 |
[Web_JSP] 05 (0) | 2022.05.23 |
[Web_JSP] 04 (0) | 2022.05.22 |
[Web_JSP] 03 (0) | 2022.05.18 |