● callback
- JS에서는 함수를 값으로 취급하기 때문에 매개변수로 전달이 가능하다.
- 함수를 리턴할 수도 있다.
● setTimeout(callback, 밀리초);
- setTimeout에 전달한 callback 함수에 write() 메소드를 사용했다면 기존에 작성했던 HTML 요소들이 사라진다.
실습(callback)
1. function에서 callback 사용하기
function mul(num1, num2, callback) {
// 외부에서 callback에 함수를 전달했다면
if(callback) {
// 매개변수로 결과를 전달해준다.
callback(num1 * num2);
}
}
function print(result) {
console.log(result);
}
mul(4, 5, print);

2. 상품명, 가격, 개수를 전달받은 뒤 전체 금액을 출력한다.
- 참고
// 1. 상품명과 가격, 개수는 A함수에서 전달받는다.
// 2. B함수에서는 상품명과 전체 금액을 전달받아서 출력한다.
// 3. A함수는 B함수를 callback함수로 전달받는다.
- 코드
(1) 함수 선언 후 사용
function pay(name, price, count, callback) {
let total = price * count;
if(callback) {
// 상품명과 전체 가격을 여기서 출력하지 않고,
// callback 함수에서 출려간다.
callback(name, total);
}
}
// pay 함수에서 연산된 결과를 total로 전달받은 뒤
function print(name, total) {
// 상품명과 total을 적절하게 출력한다.
console.log(name + " : " + total + "원");
}
pay("감자", 500, 4, print);
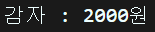
(2) 함수 선언하지 않고 바로 사용(익명)
function pay(name, price, count, callback) {
let total = price * count;
if(callback) {
// 상품명과 전체 가격을 여기서 출력하지 않고,
// callback 함수에서 출려간다.
callback(name, total);
}
}
pay("감자", 500, 4, function(name, total) {
console.log(name + " : " + total + "원");
});
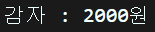
실습(setTimeout())
- 함수 선언 후 사용
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>setTimeout()</title>
</head>
<body>
<h1>지정된 시간이 흐른 뒤에 함수 실행</h1>
</body>
<script>
function writeName() {
document.write("<p>홍길동</p>");
}
writeName();
setTimeout(writeName, 3000);
</script>
</html>
- 함수 선언하지 않고 바로 사용(익명)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>setTimeout()</title>
</head>
<body>
<h1>지정된 시간이 흐른 뒤에 함수 실행</h1>
</body>
<script>
setTimeout(function() {
document.write("<p>홍길동</p>");
}, 3000);
</script>
</html>


실습(setInterval())
- 1초마다 "홍길동 " 출력하기(단, 5초 후에 종료한다.)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>setInterval()</title>
</head>
<body>
<div id="wrap"></div>
</body>
<script>
var time = setInterval(function () {
document.write("<p>홍길동</p>");
}, 1000);
setTimeout(function () {
clearInterval(time);
}, 5000);
</script>
</html>

'웹 개발 > JavaScript' 카테고리의 다른 글
[Web_JavaScript] 06 (0) | 2022.05.02 |
---|---|
[Web_JavaScript] 05 (0) | 2022.05.01 |
[Web_JavaScript] 03 (0) | 2022.04.29 |
[Web_JavaScript] 02 (0) | 2022.04.28 |
[Web_JavaScript] 01 (0) | 2022.04.27 |