● 오류
1. 예외(Exception)
- 프로그램 실행 중에 발생하는 오류 중에서 처리가 가능한 것
(1) 런타임 오류 : 실행 중에 발생하는 오류
(2) 컴파일 오류 : 컴파일 되기 전에 발생하는 오류
2. 에러(Error)
- 프로그래머가 고칠 수 없는 오류(메모리 부족)
● try-catch 문
try {
예외가 발생할 수 있는 코드;
} catch(Exception 예외객체) {
예외가 발생했을 때 수행할 코드;
}
※ 세분화
try {
예외가 발생할 수 있는 코드;
} catch(예외클래스1 예외객체) {
예외가 발생했을 때 수행할 코드;
} catch(예외클래스2 예외객체) {
예외가 발생했을 때 수행할 코드;
} ... {
} catch(Exception 예외객체) {
} finally {
}
● throws
- 예외처리를 던져버리는 키워드
- throws를 통해서 예외처리를 떠넘기면 사용하는 사람이 원하는대로 예외를 처리할 수 있다.
- 여러개의 예외는 콤마를 통해 연결하면 된다.
실습(Exception)
1. 사용자에게 인덱스번호와 숫자를 입력 받아 ar 배열 속 해당 인덱스의 요소를 숫자로 나누어 출력하는 프로그램
import java.util.InputMismatchException;
import java.util.Scanner;
public class ExceptionTest {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int[] ar = {10, 20, 30};
try {
System.out.print("인덱스 번호 입력 >> ");
int idx = sc.nextInt();
System.out.print("나눌 수 입력 >> ");
int num = sc.nextInt();
System.out.println("결과 : " + ar[idx] / num);
} catch(ArithmeticException e) {
System.out.println("0으로는 나눌 수 없음");
} catch(ArrayIndexOutOfBoundsException e) {
System.out.println("인덱스는 0부터 2까지");
} catch(InputMismatchException e) {
System.out.println("숫자만 입력하시오");
} catch(Exception e) {
e.printStackTrace();
System.out.println("알 수 없는 오류 발생");
} finally {
System.out.println("예외가 발생하든 안하든 무조건 실행되는 영역");
}
System.out.println("정상적으로 프로그램 종료됨");
}
}
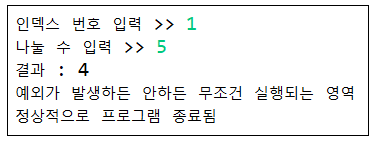
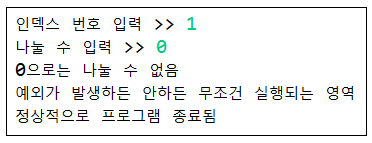

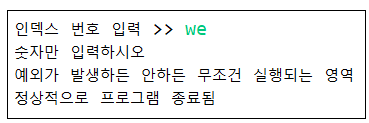
실습(throws)
1. throws를 사용한 메소드 생성
public class Dog {
public void wash(int a) throws InterruptedException, ArithmeticException {
System.out.println("1초동안 씻는 중");
Thread.sleep(1000);
System.out.println(10 / a);
System.out.println("완료");
}
}
2. Main.java에서 메소드 실행
- d.wash(5)
public class Main {
public static void main(String[] args) {
Dog d = new Dog();
try {
d.wash(5);
} catch (InterruptedException e) {
System.out.println("안녕하세요");
} catch (ArithmeticException e) {
System.out.println("안녕하세요~~");
}
}
}

- d.wash(0)
public class Main {
public static void main(String[] args) {
Dog d = new Dog();
try {
d.wash(0);
} catch (InterruptedException e) {
System.out.println("안녕하세요");
} catch (ArithmeticException e) {
System.out.println("안녕하세요~~");
}
}
}
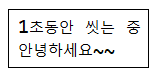