● 클래스 선언
class 클래스명 {
코드;
}
● 생성자
- 메소드와 똑같지만 리턴타입이 없다.(이미 기능이 정해져 있기 때문)
1. 기본 생성자(default constructor)
- 클래스명()
(ex) Studenet()
- 클래스 안에 생성자가 정의되어 있지 않다면 컴파일러가 알아서 기본 생성자를 만들어 준다.
- 정의된 생성자가 존재한다면 기본 생성자를 자동으로 만들어 주지 않는다.
- 직접 정의한 생성자와 기본 생성자를 동시에 사용하고 싶다면 메소드 오버로딩처럼 명시적으로 정의해주어야 한다.
2. 초기화 생성자
- 클래스명(매개변수, 매개변수, ...)
- 매개변수로부터 값을 전달받아 인스턴스 변수를 초기화 하는데에 사용할 수 있다.
● this
- 자기 자신 객체를 의미한다.
● static 제어자
- 클래스명.static변수명 or 클래스명.static메소드명()
1. static 변수(= 클래스 변수, 정적 변수)
- 인스턴스끼리 값을 공유한다.
- 모든 인스턴스가 동일하게 값을 공유해야하는 상황에서 사용한다.
2. static 메소드
- 인스턴스와는 상관없이 메소드를 사용할 때 활용한다.
(ex) Integer.parseInt("10");
- 모든 인스턴스에 대해서 동일하게 동작해야하므로 인스턴스마다 달라지는 인스턴스 변수는 사용할 수 없다.
- static 변수는 사용 가능하다.
※ 인스턴스 변수를 활용해야할 때 일반 메소드를 사용하면 매개변수 없이 인스턴스 변수를 활용할 수 있다.
실습(Class 복습)
※ 클래스 파일을 따로 생성하여 객체화 후 사용하기
- 클래스 생성
public class Student { // 필드 위에 4개의 변수와 1개의 메소드가 있다.
String name; // 멤버, 멤버 변수, 인스턴스 변수
int kor;
int eng;
int math;
//기본 생성자
public Student() { }
// 초기화 생성자
public Student(String name, int kor, int eng, int d) {
this.name = name;
this.kor = kor;
this.eng = eng;
this.math = d;
}
public void introduce() { // 멤버, 멤버 함수(= 메소드)
System.out.println("---" + this.name + " 학생정보---");
System.out.println("국어점수 : " + this.kor);
System.out.println("영어점수 : " + this.eng);
System.out.println("수학점수 : " + this.math);
}
}
- 생성한 클래스 사용
import day11.Student;
public class ClassTest {
public static void main(String[] args) {
Student bae = new Student();
Student kim = new Student("김철수", 90, 80, 70); //객체화
Student hong = new Student("홍길동", 100, 100, 100);
kim.introduce();
hong.introduce();
}
}

실습(static)
※ 클래스 파일을 따로 생성하여 객체화 후 사용하기
- 클래스 생성
public class Korean {
String name; // 인스턴스변수
int age;
static String country = "Korea"; // 모든 인스턴스가 값을 공유하는 변수(멤버, 멤버변수, 클래스변수)
public void introduce() {
System.out.println("내 이름은 " + name + "이다.");
System.out.println("내 나이는 " + age + "이다.");
}
public static void introduce(Korean k) {
System.out.println("내 이름은 " + k.name + "이다.");
System.out.println("내 나이는 " + k.age + "이다.");
}
public static void f() { // 모든 인스턴스에 동일하게 동작하는 메소드
System.out.println("static 메소드 f()가 호출됨");
// 인스턴스 변수는 인스턴스마다 다른 값을 가지므로 모든 인스턴스에 동일하게 동작해야하는 static메소드에서 사용할 수 없다.
// System.out.println(this.name); // 오류발생
System.out.println(country); //class변수는 인스턴스마다 달라지지 않기 때문에 static메소드에서 사용 가능하다.
}
}
- 생성한 클래스 사용
public class StaticTest {
public static void main(String[] args) {
Korean kim = new Korean();
Korean park = new Korean();
// static 메소드(introduce() 메소드)
kim.name = "김철수";
kim.age = 10;
Korean.introduce(kim);
// static 변수
// country는 static 변수이므로, Korean 타입의 인스턴스(kim, park)들이 값을 공유한다.
kim.country = "USA";
System.out.println(kim.country);
System.out.println(park.country);
System.out.println(Korean.country); // static 변수는 인스턴스에 따라서 값이 달라지지 않기 때문에
// 인스턴스명.static변수명 으로 쓰는 대신 class명.static변수명 으로 사용할 수 있다.
// static 메소드
kim.f();
park.f();
Korean.f();
// "10"을 int 10으로 바꿔주기만 하면 되기 때문에, 앞에 어떤 인스턴스가 오는지는 중요하지 않다.
// 따라서 static메소드로 구현되어있다.
Integer.parseInt("10");
String name = "홍길동";
name.equals("홍길동");
}
}
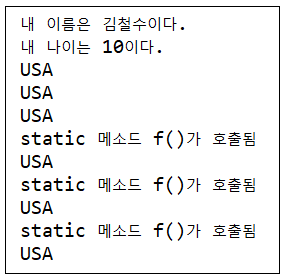