실습(Random)
import java.util.Random;
public class RandomTest {
public static void main(String[] args) {
Random r = new Random();
System.out.println(r.nextInt(5)); //nextInt(숫자) : 0부터 입력한 숫자 중 랜덤으로 하나의 정수를 가져온다.
}
}

실습(코인노래방 프로그램 만들기)
1. 금액 입력
Scanner sc = new Scanner(System.in);
int price = 0; // 사용자 금액을 입력받을 변수
int price2 = 500;
System.out.print("금액을 입력해주세요 >> ");
price = sc.nextInt();
if(price < price2) {
System.out.println(price2 + "원 이상의 금액을 넣어주세요");
continue;
}
2. 부를 수 있는 노래 곡 수와 잔돈 출력하기
songCnt += (price / price1) * songCntForPrice1
+ (price % price1)/price2 * songCntForPrice2;
int rest = price % price1 % price2;
System.out.println("부를 수 있는 노래 곡 수 :" + songCnt);
if(rest != 0) {
System.out.println("잔돈 "+ rest +"원이 반환되었습니다");
}
3. 노래부르기
- 노래 곡 수가 0곡인데 노래부르기를 클릭했다면 금액을 먼저 입력해주세요 출력한 후 메인 메뉴로 이동시키기
if(songCnt >= 1) {
totalSongCnt++;
songCnt--;
System.out.print("간주 점프??(1. yes 2. no) >> ");
choice = sc.nextInt();
if(choice == 2) {
System.out.println("간주중");
}
int score = r.nextInt(100)+1;
totalScore += score;
System.out.println("당신의 점수는 "+score+"점");
switch(score / 10) {
case 10:
case 9:
System.out.println("가수하세요");
break;
case 8:
case 7:
System.out.println("노력하세요");
break;
default:
System.out.println("오지마세요");
}
System.out.println("남은 노래 곡 수 : "+ songCnt);
} else {
System.out.println("금액을 먼저 입력해 주세요");
}
4. 내노래 실력 보기
- 사용자가 지금까지 불렀던 노래의 평균 점수(지금까지 받았던 점수의 총합 / 지금까지 불렀던 노래 곡 수)
if(totalSongCnt == 0) {
System.out.println("노래를 먼저 불러주세요");
continue;
}
System.out.println("당신의 점수 평균 : " + totalScore / (double)totalSongCnt);
} else {
System.out.println("다시 입력해주세요");
}
5. 결과

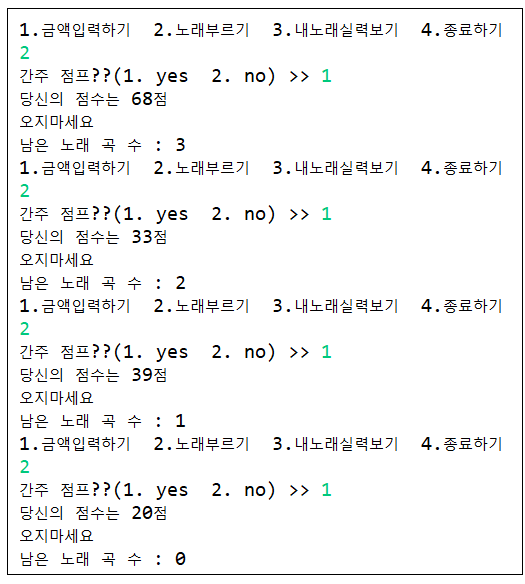


※ 전체 코드
import java.util.Random;
import java.util.Scanner;
public class Noraebang {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Random r = new Random();
int choice = 0; // 사용자 선택을 입력받을 변수
int price = 0; // 사용자 금액을 입력받을 변수
int songCnt = 0;// 부를 수 있는 노래 곡수를 담아줄 변수
int totalSongCnt = 0;
int totalScore = 0;
// 가격이 변동되는 경우 유지 보수를 위해 변수로 선언해준다.
int price1 = 1000;
int price2 = 500;
int songCntForPrice1 = 3;
int songCntForPrice2 = 1;
System.out.println("★코인노래방★");
while(true) {
System.out.println("1.금액입력하기 2.노래부르기 3.내노래실력보기 4.종료하기");
choice = sc.nextInt();
if(choice == 4) {
System.out.println("종료종료");
break;
} else if(choice == 1) {
// 금액 입력 기능
System.out.print("금액을 입력해주세요 >> ");
price = sc.nextInt();
if(price < price2) {
System.out.println(price2+"원 이상의 금액을 넣어주세요");
continue;
}
// 부를 수 있는 노래 곡 수와 잔돈 출력하기
songCnt += (price / price1) * songCntForPrice1
+ (price % price1)/price2 * songCntForPrice2;
int rest = price % price1 % price2;
System.out.println("부를 수 있는 노래 곡 수 :" + songCnt);
if(rest != 0) {
System.out.println("잔돈 "+ rest +"원이 반환되었습니다");
}
} else if(choice == 2) {
// 노래부르기기능
// 노래 곡 수가 0곡인데 노래부르기를 클릭했다면
// 금액을 먼저 충전해주세요 출력한 후 메인 메뉴로 이동시키기
if(songCnt >= 1) {
totalSongCnt++;
songCnt--;
System.out.print("간주 점프??(1. yes 2. no) >> ");
choice = sc.nextInt();
if(choice == 2) {
System.out.println("간주중");
}
int score = r.nextInt(100)+1;
totalScore += score;
System.out.println("당신의 점수는 "+score+"점");
switch(score / 10) {
case 10:
case 9:
System.out.println("가수하세요");
break;
case 8:
case 7:
System.out.println("노력하세요");
break;
default:
System.out.println("오지마세요");
}
System.out.println("남은 노래 곡 수 : "+ songCnt);
}else {
System.out.println("금액을 먼저 입력해 주세요");
}
}else if(choice == 3) {
// 내노래 실력 보기 기능
// 사용자가 지금까지 불렀던 노래의 평균 점수
// 지금까지 받았던 점수의 총합 / 지금까지 불렀던 노래 곡수
if(totalSongCnt == 0) {
System.out.println("노래를 먼저 불러주세요");
continue;
}
System.out.println("당신의 점수 평균 : " + totalScore / (double)totalSongCnt);
} else {
System.out.println("다시 입력해주세요");
} //조건문 닫는 중괄호
} //while문 닫는 중괄호
}
}