실습(메소드)
- 동물 키우기 프로그램
1. 필요한 변수 선언하기
String name; // 이름
int age; // 나이
String feed; // 먹이 종류
int feedCount; // 먹이 개수
int life; // 생명
※ 생성자 생성하기
public Animal() {;}
public Animal(String name, int age, String feed, int feedCount, int life) {
this.name = name;
this.age = age;
this.feed = feed;
this.feedCount = feedCount;
this.life = life;
}
2. 먹기(먹이 개수 1 감소, 생명 1 증가)
boolean eat() {
if(feedCount < 1) { // 먹이 개수가 0개일 때
return false;
} else { // 먹이 개수가 1개 이상일 때
feedCount--;
life++;
return true;
}
}
3. 잠자기(생명 1 증가)
void sleep() {
life++;
}
4. 산책하기(생명 1 감소, 랜덤 문제 출제)
Quiz walk(Quiz[] arQuiz) {
life--;
int rating = 10; // 10%(총 100칸)
int[] arData = new int[10]; // 총 10칸
Random r = new Random();
Quiz quiz = new Quiz();
for (int i = 0; i < rating / 10; i++) { // rating과 arData의 칸 수를 동일하게 설정해야 하므로
// rating / 10을 해서 rating을 10칸으로 만들어준다.
arData[i] = 1;
}
if(arData[r.nextInt(arData.length)] == 1) {//10%
while(true) {
// 랜덤한 문제를 골라서 quiz에 담아준다.
quiz = arQuiz[r.nextInt(arQuiz.length)];
// 잭팟 문제라면 탈출한다.
if(quiz.jackpot) {break;}
}
} else {//90%
while(true) {
// 랜덤한 문제를 골라서 quiz에 담아준다.
quiz = arQuiz[r.nextInt(arQuiz.length)];
// 일반 문제라면 탈출한다.
if (!quiz.jackpot) {break;}
}
}
return quiz;
}
5. Quiz.java에 생성자 생성
public class Quiz {
String question;
int answer;
int feedCount;
boolean jackpot;
public Quiz() {;}
public Quiz(String question, int answer, int feedCount) {
this.question = question;
this.answer = answer;
this.feedCount = feedCount;
}
public Quiz(String question, int answer, int feedCount, boolean jackpot) {
this.question = question;
this.answer = answer;
this.feedCount = feedCount;
this.jackpot = jackpot;
}
}
6. main 메소드 안에서 실행
- 필요한 변수 및 배열 선언
Zoo zoo = new Zoo();
Animal[] animals = new Animal[3];
Animal animal = null;
animals[0] = new Animal("기린", 10, "풀", 5, 5);
animals[1] = new Animal("거북이", 15, "해초", 8, 2);
animals[2] = new Animal("여우", 3, "고기", 4, 6);
Quiz[] arQuiz = {
new Quiz("Q. 다음 중 프로그래밍 언어가 아닌 것은?\n1. JAVA\n2. C언어\n3. 파이썬\n4. 망둥어", 4, 2),
new Quiz("Q. 다음 중 JAVA에 없는 챕터는?\n1. 변수\n2.클래스\n3. 메소드\n4. 포인터", 4, 100, true),
new Quiz("Q. 다음 중 JAVA 기본 자료형이 아닌 것은?\n1. int\n2.boolean\n3. char\n4. String", 4, 50, true)
};
Quiz quiz = null; // 주소값을 null로 초기화
Scanner sc = new Scanner(System.in);
String menuMsg = "캐릭터 선택하기";
String actionMsg = "1. 먹기\n2. 잠자기\n3. 산책하기\n4. 작별인사하기";
int charNum = 0; // 사용자가 선택한 캐릭터 번호를 담아준다.
int actionChoice = 0; // 사용자가 선택한 행동 번호를 담아준다.
int answer = 0; // 사용자가 선택한 퀴즈의 답을 담아준다.
- 먹기(먹이 개수가 0개라면 먹기 실패)
if(animal.eat()) { // animal.eat() : true
System.out.println(animal.name + "(이)가" + animal.feed + "(을)를 먹는 중");
System.out.println("현재 " + animal.feed + " 개수 : " + animal.feedCount + "개");
System.out.println("현재 체력 : " + animal.life);
break;
}
System.out.println(animal.feed + "(이)가 없습니다. 산책하러 가보세요.");
- 잠자기(Thread.sleep()를 사용해서 3초에 생명 1이 증가하도록 구현)
// 소스코드 간결화
void sleep(Animal animal) {
System.out.print("자는 중");
for (int j = 0; j < 3; j++) { // 3초 동안
try {Thread.sleep(1000);} catch (InterruptedException e) {;}
System.out.print(".");
}
System.out.println();
animal.sleep(); // Animal.java의 sleep() 메소드 사용
System.out.println("생명이 1 증가했습니다.");
System.out.println(animal.name + "의 생명 : " + animal.life);
}
- 산책하기(맞출 시 먹이 2개 획득 & 틀릴 시 생명 1개 감소, 잭팟을 구현하여 낮은 확률로 많은 먹이를 얻을 수 있도록 구현)
if(animal.life > 1) {
quiz = animal.walk(arQuiz);
if(quiz.jackpot) {
System.out.println("-----잭팟-----");
}
System.out.println(quiz.question);
answer = sc.nextInt();
// 정답일 경우
if(quiz.answer == answer) {
animal.feedCount += quiz.feedCount;
System.out.println("정답입니다.");
System.out.println("먹이를 " + quiz.feedCount +"개 획득했습니다.");
System.out.println("현재 " + animal.feed +" 개수 : " + animal.feedCount);
break;
}
// 오답일 경우
System.out.println("오답입니다. 다음에 다시 도전해보세요.");
animal.life--;
System.out.println("현재 체력 : " + animal.life);
if(animal.life == 0) {
zoo.sleep(animal); // Zoo.java의 sleep()메소드를 사용
}
} else {
System.out.println("남은 체력이 없습니다. 잠을 자고 오세요.");
}
※ 전체 코드
import java.util.Scanner;
public class Zoo {
void sleep(Animal animal) {
System.out.print("자는 중");
for (int j = 0; j < 3; j++) {
try {Thread.sleep(1000);} catch (InterruptedException e) {;}
System.out.print(".");
}
System.out.println();
animal.sleep();
System.out.println("생명이 1 증가했습니다.");
System.out.println(animal.name + "의 생명 : " + animal.life);
}
public static void main(String[] args) {
Zoo zoo = new Zoo();
Animal[] animals = new Animal[3];
Animal animal = null;
animals[0] = new Animal("기린", 10, "풀", 5, 5);
animals[1] = new Animal("거북이", 15, "해초", 8, 2);
animals[2] = new Animal("여우", 3, "고기", 4, 6);
Quiz[] arQuiz = {
new Quiz("Q. 다음 중 프로그래밍 언어가 아닌 것은?\n1. JAVA\n2. C언어\n3. 파이썬\n4. 망둥어", 4, 2),
new Quiz("Q. 다음 중 JAVA에 없는 챕터는?\n1. 변수\n2.클래스\n3. 메소드\n4. 포인터", 4, 100, true),
new Quiz("Q. 다음 중 JAVA 기본 자료형이 아닌 것은?\n1. int\n2.boolean\n3. char\n4. String", 4, 50, true)
};
Quiz quiz = null;
Scanner sc = new Scanner(System.in);
String menuMsg = "캐릭터 선택하기";
String actionMsg = "1. 먹기\n2. 잠자기\n3. 산책하기\n4. 작별인사하기";
int charNum = 0;
int actionChoice = 0;
int answer = 0;
while(true) {
int i = 0;
System.out.println(menuMsg);
for (i = 0; i < animals.length; i++) {
System.out.println(i + 1 + ". " + animals[i].name);
}
System.out.println(i + 1 + ". 나가기");
charNum = sc.nextInt();
if(charNum == i + 1) {break;}
animal = animals[charNum - 1];
while(true) {
System.out.println(actionMsg);
actionChoice = sc.nextInt();
if(actionChoice == 4) {break;}
switch (actionChoice) {
case 1: // 먹기
if(animal.eat()) {
System.out.println(animal.name + "(이)가" + animal.feed + "(을)를 먹는 중");
System.out.println("현재 " + animal.feed + " 개수 : " + animal.feedCount + "개");
System.out.println("현재 체력 : " + animal.life);
break;
}
System.out.println(animal.feed + "(이)가 없습니다. 산책하러 가보세요.");
break;
case 2: // 잠자기
zoo.sleep(animal);
break;
case 3: // 산책하기
if(animal.life > 1) {
quiz = animal.walk(arQuiz);
if(quiz.jackpot) {
System.out.println("-----잭팟-----");
}
System.out.println(quiz.question);
answer = sc.nextInt();
// 정답일 경우
if(quiz.answer == answer) {
animal.feedCount += quiz.feedCount;
System.out.println("정답입니다.");
System.out.println("먹이를 " + quiz.feedCount +"개 획득했습니다.");
System.out.println("현재 " + animal.feed +" 개수 : " + animal.feedCount);
break;
}
// 오답일 경우
System.out.println("오답입니다. 다음에 다시 도전해보세요.");
animal.life--;
System.out.println("현재 체력 : " + animal.life);
if(animal.life == 0) {
zoo.sleep(animal);
}
} else {
System.out.println("남은 체력이 없습니다. 잠을 자고 오세요.");
}
break;
} // switch문 종료
} // while문 종료
} // while문 종료
}
}
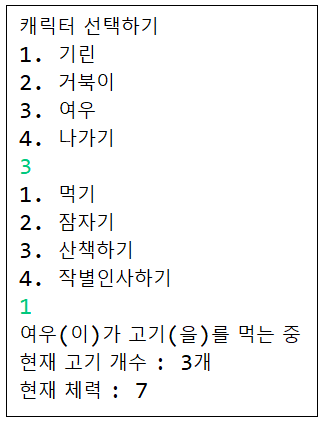
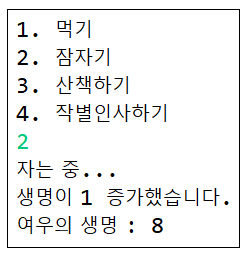
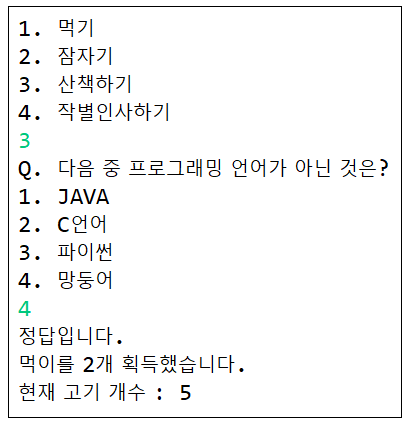
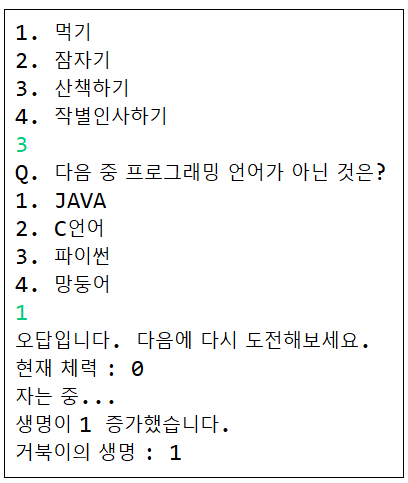
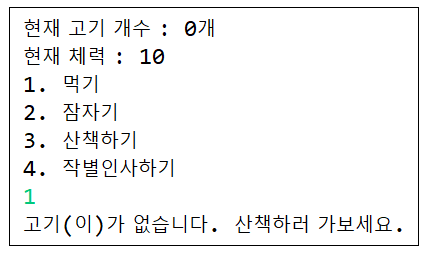
'ETC > 복습' 카테고리의 다른 글
[복습_JAVA] 23 (0) | 2022.09.07 |
---|---|
[복습_JAVA] 22 (0) | 2022.09.06 |
[복습_JAVA] 20 (0) | 2022.09.01 |
[복습_JAVA] 19 (0) | 2022.08.30 |
[복습_JAVA] 18 (0) | 2022.08.28 |