● Date 객체
var 객체명 = new Date();
● Date 객체의 주요 메소드
1. getFullYear(): 4자리 년도
2. getMonth(): 0~11사이의 정수(0: 1월, 1: 2월, ..., 11: 12월)
3. getDate(): 한 달 내의 날짜(28~31)
4. getDay(): 한 주 내 요일(0: 일요일, 1: 월요일, ..., 6: 토요일)
5. getHours(): 0~23사이의 정수
6. getMinutes(): 0~59사이의 정수
7. getSeconds(): 0~59사이의 정수
8. getMilliseconds(): 0~999사이의 정수
9. getTime(): 1970년 1월 1일 0시 0분 0초 기준 현재까지의 밀리초
10. setFullYear(year): 년도 저장
11. setMonth(month): 월 저장
12. setDate(date): 한 달 내의 날짜 값 지정
13. setHours(hour): 시간 저장
14. setMinutes(minute): 분 저장
15. setSeconds(second): 초 저장
16. setMilliseconds(ms): 밀리초 저장
17. setTime(t): 밀리초 단위인 t값으로 시간 저장
실습(파일 입출력(1))
0. 회원 정보 저장
let file = require('fs');
let user = new Object();
user.name = "홍길동";
user.id = "hong1234";
user.pw = "1234";
1. 파일 출력(writeFile())
- 코드
let userJSON;
userJSON = JSON.stringify(user);
file.writeFile('day05/user.json', userJSON, 'utf-8', function(e){
if(e){
console.log(e);
}else{
console.log("파일 출력 성공");
}
});
※ 결과 : user.json 파일 생성

2. 파일 입력(readFile())
file.readFile('day05/user.json', 'utf-8', function(e, content){
let user = JSON.parse(content);
console.log(user.name);
console.log('아이디: %s', user.id);
console.log(user.pw);
});
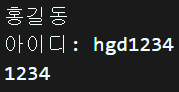
실습(파일 입출력(2))
- 문제
// 상품명, 가격, 재고를 JS 객체에 프로퍼티로 담는다.
// JSON형식으로 변환한 뒤, day05/product.json으로 출력한다.
// day05/product.json에 있는 JSON 형식을 JS Object타입으로 변환한다.
// 각 프로퍼티를 출력한다.
- 코드
let file = require('fs');
let product = new Object();
let productJSON;
product.name = "굴렁쇠";
product.price = 4900;
product.stock = 4;
productJSON = JSON.stringify(product);
file.writeFile('day05/product.json', productJSON, 'utf-8', function(e) {
if(e) {
console.log(e);
} else {
console.log("파일 출력 성공");
}
});
// day05/product.json에 있는 JSON 형식을 JS Object타입으로 변환한다.
// 각 프로퍼티를 출력한다.
file.readFile('day05/product.json', 'utf-8', function(e, content) {
let product = JSON.parse(content);
console.log(product.name);
console.log(product.price);
console.log(product.stock);
});
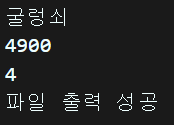
실습(Date 객체(1))
var now = new Date(); // 현재 시간
console.log(now);
console.log(now.getFullYear()); // 올해 년도

실습(Date 객체(2))
- 문제
// 방문 시간이
// 홀수일 때 pink로 배경색 변경
// 짝수일 때 orange로 배경색 변경
- 코드
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>코어객체 - Date()</title>
</head>
<body>
<h3>방문 시간에 따라 변하는 배경</h3>
<hr>
</body>
<script>
var now = new Date();
document.body.style.backgroundColor = now.getSeconds() % 2 == 0 ? "orange" : "pink";
</script>
</html>

실습(타이머)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>타이머</title>
</head>
<body>
<div id='clock'></div>
</body>
<script>
setInterval(function(){
let now = new Date();
document.getElementById('clock').innerHTML = "<p>" + now + "</p>";
}, 1000);
</script>
</html>

실습(Math)
- 구구단 게임
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Math를 이용한 구구단 게임</title>
</head>
<body>
<h3>Math를 이용한 구구단 게임</h3>
<hr>
</body>
<script>
// Math.floor(Math.random() * n): 0 ~ n-1의 난수
// Math.random() : 1보다 작은 0 ~ 1 사이의 실수를 리턴한다.
function getRandomInt(){
// 마지막에 +1을 하여 1 ~ 9 사이의 수가 리턴되도록 한다.
return Math.floor(Math.random() * 9) + 1;
}
let question = getRandomInt() + "*" + getRandomInt();
let answer = eval(question);
let myAnswer = prompt(question);
if(myAnswer == null || isNaN(parseInt(myAnswer))){
document.write("연습 종료");
} else{
if(answer == myAnswer){
document.write("<h1>정답!</h1>");
} else{
document.write("<h1 style='color:red; font-size:1px;'>오답..</h1>");
}
}
</script>
</html>
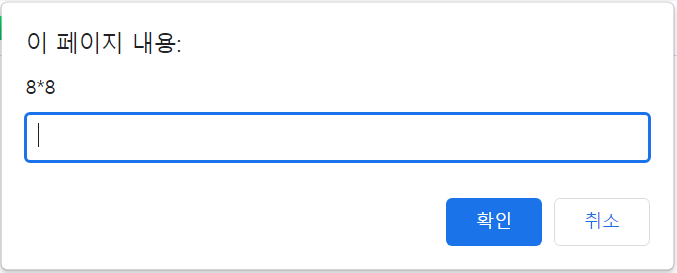
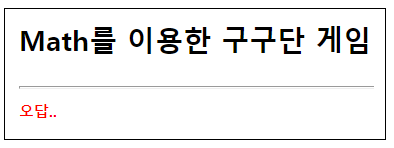

'웹 개발 > JavaScript' 카테고리의 다른 글
[Web_JavaScript] 10 (0) | 2022.05.06 |
---|---|
[Web_JavaScript] 09 (0) | 2022.05.05 |
[Web_JavaScript] 07 (0) | 2022.05.03 |
[Web_JavaScript] 06 (0) | 2022.05.02 |
[Web_JavaScript] 05 (0) | 2022.05.01 |