● 컬렉션 프레임워크(Collection Framework)
- Collection
(3) Map
- key와 value 한 쌍으로 짝지어져 저장되는 형태 (인덱스번호를 마음대로 설정한다.)
- HashMap, TreeMap, ... → 사용 방법은 같지만 구현 방식에서 차이가 난다.
실습(HashMap)
0. size(), isEmpty()
import java.util.HashMap;
public class HashMapTest {
public static void main(String[] args) {
HashMap<String, Integer> scores = new HashMap<String, Integer>();
System.out.println(scores.size());
System.out.println(scores.isEmpty());
}
}

1. put(key, value) : key 값 추가
import java.util.HashMap;
public class HashMapTest {
public static void main(String[] args) {
HashMap<String, Integer> scores = new HashMap<String, Integer>();
// put(key, value) : key 값이 없던 key라면 값 추가
// key는 중복될 수 없고, value는 중복될 수 있다.
scores.put("국어", 80);
scores.put("영어", 70);
scores.put("수학", 100);
System.out.println(scores);
}
}

2. put(key, value) : key 값 수정
import java.util.HashMap;
public class HashMapTest {
public static void main(String[] args) {
HashMap<String, Integer> scores = new HashMap<String, Integer>();
scores.put("국어", 80);
scores.put("영어", 70);
scores.put("수학", 100);
// put(key, value) : key가 존재하던 key라면 값 수정
scores.put("국어", 100);
System.out.println(scores);
}
}

3. get(key)
import java.util.HashMap;
public class HashMapTest {
public static void main(String[] args) {
HashMap<String, Integer> scores = new HashMap<String, Integer>();
scores.put("국어", 80);
scores.put("영어", 70);
scores.put("수학", 100);
// get(key) : key에 해당하는 value를 return
System.out.println(scores.get("국어"));
System.out.println(scores.get("수학"));
System.out.println(scores.get("영어"));
}
}

4. remove(key)
import java.util.HashMap;
public class HashMapTest {
public static void main(String[] args) {
HashMap<String, Integer> scores = new HashMap<String, Integer>();
scores.put("국어", 80);
scores.put("영어", 70);
scores.put("수학", 100);
// remove(key) : 삭제
scores.remove("수학");
System.out.println(scores);
System.out.println(scores.size());
}
}

5. keySet()
import java.util.HashMap;
public class HashMapTest {
public static void main(String[] args) {
HashMap<String, Integer> scores = new HashMap<String, Integer>();
scores.put("국어", 80);
scores.put("영어", 70);
scores.put("수학", 100);
// key들이 들어있는 Set가 return된다.
System.out.println(scores.keySet());
for (String key : scores.keySet()) {
System.out.println(key);
System.out.println(scores.get(key));
}
}
}
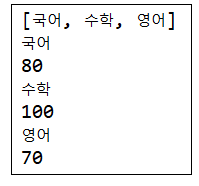
6. values()
import java.util.HashMap;
public class HashMapTest {
public static void main(String[] args) {
HashMap<String, Integer> scores = new HashMap<String, Integer>();
scores.put("국어", 80);
scores.put("영어", 70);
scores.put("수학", 100);
// values() : value들이 들어있는 반복 가능 컬렉션을 return한다.
System.out.println(scores.values());
for (Integer score : scores.values()) {
System.out.println(score);
}
}
}
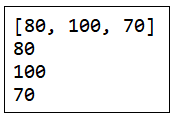
7. entrySet()
import java.util.HashMap;
import java.util.Map.Entry;
public class HashMapTest {
public static void main(String[] args) {
HashMap<String, Integer> scores = new HashMap<String, Integer>();
scores.put("국어", 80);
scores.put("영어", 70);
scores.put("수학", 100);
// entrySet() : set 안에 Entry 객체가 들어있다.
// Entry는 key와 value 한 쌍의 정보를 담고 있다.
System.out.println(scores.entrySet());
for (Entry<String, Integer> ent : scores.entrySet()) {
System.out.println(ent.getKey()); // Entry 객체 내의 key를 return
System.out.println(ent.getValue()); // Entry 객체 내의 value를 return
}
}
}
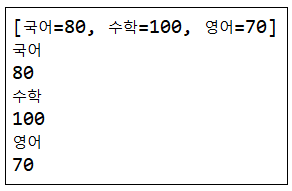